Examples
Here you will find a set of example figures with the code that generated them.
In order for the examples to work on your computer, you need to have a Tango device server running. The following section explains how to do this.
Setup
The device server used for the examples can be obtained here
.
In order for the examples to work as they are provided a TaurusTest device must be created and running with the following configuration:
Server (ServerName/Instance):
TaurusTest/taurustest
Class:
TaurusTest
Devices:
sys/taurustest/1
You can easily configure it from Jive by going to Edit->Create server and type the above parameters in the dialog that pops up.
Common
For the sake of simplicity the code presented below (except for the first example) does not include the following header and footer code lines:
header:
1import sys
2from taurus.external.qt import Qt
3from taurus.qt.qtgui.application import TaurusApplication
4
5app = TaurusApplication(sys.argv, cmd_line_parser=None)
6panel = Qt.QWidget()
7layout = Qt.QHBoxLayout()
8panel.setLayout(layout)
footer:
panel.show()
sys.exit(app.exec_())
You must prepend and append the above code in order for the examples to work properly.
Display attribute value
Displaying a tango attribute value in a GUI is easy with taurus and
display.TaurusLabel
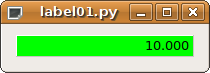
code:
1import sys
2from taurus.external.qt import Qt
3from taurus.qt.qtgui.application import TaurusApplication
4
5app = TaurusApplication(sys.argv, cmd_line_parser=None,)
6panel = Qt.QWidget()
7layout = Qt.QHBoxLayout()
8panel.setLayout(layout)
9
10from taurus.qt.qtgui.display import TaurusLabel
11w = TaurusLabel()
12layout.addWidget(w)
13w.model = 'sys/taurustest/1/position'
14
15panel.show()
16sys.exit(app.exec_())
not much code to write, but… boring!
Display attribute value with label
Let’s spice it up a bit: add the tango label for the position attribute so it looks something like this:
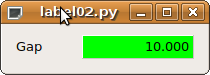
code:
1from taurus.qt.qtgui.display import TaurusLabel
2w1, w2 = TaurusLabel(), TaurusLabel()
3layout.addWidget(w1)
4layout.addWidget(w2)
5w1.model, w1.bgRole = 'sys/taurustest/1/position#label', ''
6w2.model = 'sys/taurustest/1/position'
Much better indeed!
Display attribute value with label and separate units
And little bit more… add the units.
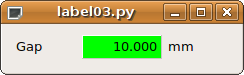
code:
1from taurus.qt.qtgui.container import TaurusWidget
2from taurus.qt.qtgui.display import TaurusLabel
3
4w1, w2, w3 = TaurusLabel(), TaurusLabel(), TaurusLabel()
5layout.addWidget(w1)
6layout.addWidget(w2)
7layout.addWidget(w3)
8w1.model, w1.bgRole = 'sys/taurustest/1/position#label', ''
9w2.model = 'sys/taurustest/1/position#rvalue.magnitude'
10w3.model, w3.bgRole = 'sys/taurustest/1/position#rvalue.units', ''
Nice isn’t it?
Interactively display attribute
Humm… Now suppose the user wants to change this value. input.TaurusValueLineEdit
does this job well (and so does input.TaurusValueSpinBox
and
input.TaurusWheelEdit
😃 )

With TaurusValueLineEdit
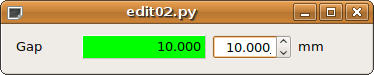
With TaurusValueSpinBox
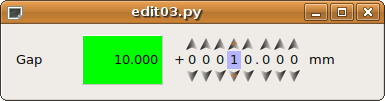
With TaurusWheelEdit
code:
1from taurus.qt.qtgui.display import TaurusLabel
2from taurus.qt.qtgui.input import TaurusValueLineEdit, TaurusValueSpinBox, TaurusWheelEdit
3
4w1 = TaurusLabel()
5w2 = TaurusLabel()
6w3 = TaurusValueLineEdit() # or TaurusValueSpinBox or TaurusWheelEdit
7w4 = TaurusLabel()
8layout.addWidget(w1)
9layout.addWidget(w2)
10layout.addWidget(w3)
11layout.addWidget(w4)
12w1.model, w1.bgRole = 'sys/taurustest/1/position#label', ''
13w2.model = 'sys/taurustest/1/position'
14w3.model = 'sys/taurustest/1/position'
15w4.model, w4.bgRole = 'sys/taurustest/1/position#rvalue.units', ''
Now it seems a little bit more useful, doesn’t it?
A higher level of abstraction: forms
Now let’s say you want to display not only one but a dozen attributes… the
programming becomes quite tedious. Taurus provides a higher level of
abstraction: the panel.TaurusForm
.
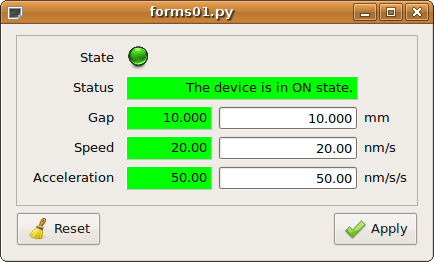
code:
1from taurus.qt.qtgui.panel import TaurusForm
2
3panel = TaurusForm()
4props = [ 'state', 'status', 'position', 'velocity', 'acceleration' ]
5model = [ 'sys/taurustest/1/%s' % p for p in props ]
6panel.setModel(model)
…and don’t worry: panel.TaurusForm
properly aligns the labels,
manages the apply buttons and most important, it automagically decides which are the most appropriate
widgets to use depending on the kind of attribute (you do not need to worry
about whether the attribute is a scalar or a spectrum; or if it is read-only or
writable; a boolean or a float, etc).
I specially enjoyed this one… let’s see what’s next!
Customizing forms
TaurusForm is highly customizable. This example shows how you can change the default widget for some attributes according to the user needs.
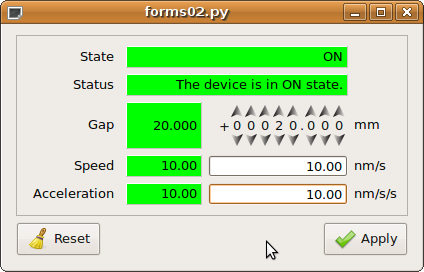
code:
1from taurus.qt.qtgui.panel import TaurusForm
2from taurus.qt.qtgui.display import TaurusLabel
3
4panel = TaurusForm()
5props = [ 'state', 'status', 'position', 'velocity', 'acceleration' ]
6model = [ 'sys/taurustest/1/%s' % p for p in props ]
7panel.setModel(model)
8panel[0].readWidgetClass = TaurusLabel # you can provide an arbitrary class...
9panel[2].writeWidgetClass = 'TaurusWheelEdit' # ...or, if it is a Taurus class you can just give its name
A little configuration goes a long way!
You can also change style properties like font size, borders, colors…
code:
1from taurus.qt.qtgui.panel import TaurusForm
2
3panel = TaurusForm()
4props = [ 'state', 'status', 'position', 'velocity', 'acceleration' ]
5model = [ 'sys/taurustest/1/%s' % p for p in props ]
6panel.setModel(model)
7panel.setStyleSheet("font-size: 40px;")
The recommended way to change the style is using the setStyleSheet method on the TaurusForm (or any other widget). However, if you want to apply a style on a specific widget inside a TaurusValue, you need to use Qt methods as Taurus overrides some of the subwidget styles.
code:
1from taurus.qt.qtgui.panel import TaurusForm
2
3panel = TaurusForm()
4props = [ 'state', 'status', 'position', 'velocity', 'acceleration' ]
5model = [ 'sys/taurustest/1/%s' % p for p in props ]
6panel.setModel(model)
7for row in w:
8 row.readWidget().setFont(Qt.QFont("Sans Serif",40))
Synoptics one-o-one
Todo
put a jdraw synoptics here
Let’s go graphical
The plot widgets are provided by the taurus_pyqtgraph plugin.
Simple plotting of various spectrum attributes
Say you want to plot two 1D attributes and watch them changing on-line?
The taurus_pyqtgraph plugin provides a very complete
widget: taurus_pyqtgraph.TaurusPlot
code:
from taurus_pyqtgraph import TaurusPlot
panel = TaurusPlot()
model = ['sys/taurustest/1/abscissas', 'sys/taurustest/1/curve']
panel.setModel(model)
Scatter plots (Y vs X plots)
In the former example each element of the spectrum attributes, was assigned its position index as the x-value (i.e., the “abscissas” attribute was plotted as a spectrum). But, what if you want to create a scatter plot where you want to read the x-values from one attribute and the y-values from another? Then set the attributes in a tuple, where the first element is the x-values and the second one the y-values.
code:
from taurus_pyqtgraph import TaurusPlot
panel = TaurusPlot()
model = [('sys/taurustest/1/abscissas', 'sys/taurustest/1/curve')]
panel.setModel(model)
Note that now the sys/taurustest/1/abscissas attribute is being used as x-values instead of being considered as another spectrum to plot like before.
Plotting data that is not an attribute
A taurus_pyqtgraph.TaurusPlot
widget is a pyqtgraph.PlotWidget
You may use the standard API from PyQtGraph for plotting other data:
code:
1from taurus_pyqtgraph import TaurusPlot
2import pyqtgraph as pg
3import numpy
4
5panel = TaurusPlot()
6model = [('sys/taurustest/1/abscissas,sys/taurustest/1/curve')]
7panel.setModel(model)
8
9c1 = pg.PlotDataItem(name="pg item", pen="b", fillLevel=0, brush="c")
10c1.setData(numpy.linspace(0, 2, 250))
11panel.addItem(c1)
Plotting Trends
Many times we are interested in showing how a scalar attribute evolves with
time. A close-cousin of the TaurusPlot called
taurus_pyqtgraph.TaurusTrend
is here to help you:
code:
from taurus.qt.qtgui.plot import TaurusTrend
panel = TaurusTrend()
model = ['sys/taurustest/1/position']
panel.setModel(model)
Note: if you pass a model that is a 1D attribute (instead of a scalar), TaurusTrend will interpret it as a collection of scalar values and will plot a separate trend line for each.
Even higher level: creating a TaurusGui
taurusgui.TaurusGui
provides very convenient way of creating
feature-rich and very configurable GUIs by using existing widgets as “panels”.
TaurusGuis can be created via a wizard application (no programming at all!) with
a few clicks. You can try it out by running:
taurus newgui
For more details and tricks regarding TaurusGui, check this.