TaurusGui
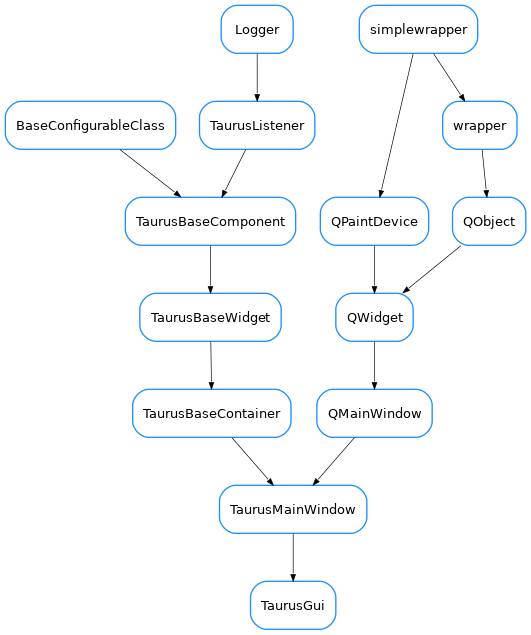
- class TaurusGui(parent=None, confname=None, configRecursionDepth=None, settingsname=None)[source]
This is main class for constructing the dynamic GUIs. TaurusGui is a specialised TaurusMainWindow which is able to handle “panels” and load configuration files. There are several ways of using TaurusGui. In the following we will give 3 examples on how to create a simple GUI called “MyGui” which contains one panel called “Foo” and consisting of a QWidget:
Example 1: use declarative configuration files.
You can create a purely declarative configuration file to be interpreted by the standard taurusgui script:
from taurus.qt.qtgui.taurusgui.utils import PanelDescription GUI_NAME = 'MyGui' panel = PanelDescription('Foo', classname='taurus.external.qt.Qt.QWidget')
Note that this just a very simple example. For a much richer one, see the
taurus.qt.qtgui.taurusgui.conf.tgconf_example01
Example 2: do everything programmatically.
A stand-alone python script that launches the gui when executed. No configuration file is used here. Panels and other components are added programatically:
if __name__ == '__main__': from taurus.qt.qtgui.application import TaurusApplication from taurus.qt.qtgui.taurusgui import TaurusGui from taurus.external.qt import Qt app = TaurusApplication(cmd_line_parser=None, app_name='MyGui') gui = TaurusGui() panel = Qt.QWidget() gui.createPanel(panel, 'Foo') gui.show() app.exec_()
Example 3: mixing declarative and programmatic ways
It is also possible to create a stand-alone python script which loads itself as a configuration file. In this way you can add things programmatically and at the same time use the declarative way:
GUI_NAME = 'MyGui' # <-- declarative! if __name__ == '__main__': from taurus.qt.qtgui.application import TaurusApplication from taurus.qt.qtgui.taurusgui import TaurusGui from taurus.external.qt import Qt app = TaurusApplication(cmd_line_parser=None) gui = TaurusGui(confname=__file__) panel = Qt.QWidget() gui.createPanel(panel, 'Foo') # <-- programmatic! gui.show() app.exec_()
Import from
taurus.qt.qtgui.taurusgui
as:from taurus.qt.qtgui.taurusgui import TaurusGui
- APPLETS_TOOLBAR_ENABLED = True
Whether to show the applets toolbar
- IMPLICIT_ASSOCIATION = '__[IMPLICIT]__'
- PANELS_MENU_ENABLED = True
Whether to show user actions related to shared data connections
- QUICK_ACCESS_TOOLBAR_ENABLED = True
Whether to add the Quick access Toolbar (empty by default)
- SelectedInstrument
- createCustomPanel(paneldesc=None)[source]
Creates a panel from a Panel Description and sets it as “custom panel”.
- Parameters:
paneldesc (PanelDescription) – description of the panel to be created
See also
- createInstrumentsFromPool(macroservername)[source]
Creates a list of instrument panel descriptions by gathering the info from the Pool. Each panel is a TaurusForm grouping together all those elements that belong to the same instrument according to the Pool info
- Returns:
- Return type:
list<PanelDescription>
- createMainSynoptic(synopticname)[source]
Creates a synoptic panel and registers it as “SelectedInstrument” reader and writer (allowing selecting instruments from synoptic
- createPanel(widget, name, floating=False, registerconfig=True, custom=False, permanent=False, icon=None, instrumentkey=None)[source]
Creates a panel containing the given widget.
- Parameters:
wiget (QWidget) – the widget to be contained in the panel
name (str) – the name of the panel. It will be used in tabs as well as for configuration
floating (bool) – whether the panel should be docked or floating. (see note below)
registerconfig (bool) – if True, the panel will be registered as a delegate for configuration
custom (bool) – if True the panel is to be considered a “custom panel”
permanent (bool) – set this to True for panels that need to be recreated when restoring the app
icon (QIcon) – icon for the panel
instrumentkey (str) – name of an instrument to which this panel is to be associated
- Returns:
the created panel
- Return type:
Note
On a previous version, there was a mandatory parameter called area (which accepted a Qt.DockWidgetArea or None as values) this parameter has now been substituted by the keyword argument floating. In order to provide backwards compatibility, the “floating” keyword argument stays at the same position as the old area argument and if a Qt.DockWidgetArea value is given, it will be interpreted as floating=True (while if None is passed, it will be interpreted as floating=False.
- doorNameChanged
- findPanelsInArea(area)[source]
returns all panels in the given area
- Parameters:
area (Qt.DockWidgetArea or str) – If area==’FLOATING’, the dockwidgets that are floating will be returned.
area
Warning
This method is deprecated
- getAllInstrumentAssociations()[source]
Returns the dictionary of instrument-panel associations
- Returns:
a dict whose keys are the instruments known to the gui and whose values are the corresponding associated panels (or None).
- Return type:
dict<str,str>
- getCustomWidgetMap(**kwargs)
Returns the default map used to create custom widgets by the TaurusForms belonging to this GUI
- return:
a dictionary whose keys are device type strings (i.e. see
PyTango.DeviceInfo
) and whose values are widgets to be used- rtype:
dict<str,Qt.QWidget>
See also
Deprecated since version 4.6.5: Use item factories instead
- getInstrumentAssociation(instrumentname)[source]
Returns the panel name associated to an instrument name
- loadConfiguration(confname)[source]
Reads a configuration file
- Parameters:
confname (str or None) – the name of module located in the PYTHONPATH or in the conf subdirectory of the directory in which taurusgui.py file is installed. This method will try to import <confname>. If that fails, it will try to import tgconf_<confname>. Alternatively, confname can be the path to the configuration module (not necessarily in the PYTHONPATH). confname can also be None, in which case a dummy empty module will be used.
- macroserverNameChanged
- newShortMessage
- onSelectedInstrument(instrumentname)[source]
Slot to be called when the selected instrument has changed (e.g. by user clicking in the synoptic)
- Parameters:
instrumentname (str) – The name that identifies the instrument.
- onShortMessage(msg)[source]
Slot to be called when there is a new short message. Currently, the only action taken when there is a new message is to display it in the main window status bar.
- Parameters:
msg (str) – the short descriptive message to be handled
- onShowManual(anchor=None)[source]
reimplemented from
TaurusMainWindow
to show the manual in a panel (not just a dockwidget)
- removeExternalApp(name=None)[source]
Remove the given external application from the GUI.
- Parameters:
name (str or None) – the name of the external application to be removed If None given, the user will be prompted
- removePanel(name=None)[source]
remove the given panel from the GUI.
Note
The panel; is actually removed from the current perspective. If the panel is saved in other perspectives, it should be removed from them as well.
- Parameters:
name (str or None) – the name of the panel to be removed If None given, the user will be prompted
- setAllInstrumentAssociations(associationsdict, clearExisting=False)[source]
Sets the dictionary of instrument-panel associations. By default, it keeps any existing association not present in the associationsdict.
- Parameters:
associationsdict (dict<str,str>) – a dict whose keys are the instruments names and whose values are the corresponding associated panels (or None)
clearExisting (bool) – if True, the the existing asociations are cleared. If False (default) existing associations are updated with those in associationsdict
- setCustomWidgetMap(**kwargs)
Sets the widget map that is used application-wide. This widget map will be used by default in all TaurusForm Panels belonging to this gui.
- param map:
a dictionary whose keys are device type strings (e.g. see
PyTango.DeviceInfo
) and whose values are widgets to be used- type map:
dict<str,Qt.QWidget>
See also
TaurusForm.setCustomWidgetMap()
,getCustomWidgetMap()
Deprecated since version 4.6.5: Use item factories instead
- setFocusToPanel(panelname)[source]
Method that sets a focus for panel passed via an argument
- Parameters:
panelname (str) – The name that identifies the panel. This name must be unique within the panels in the GUI.
- setInstrumentAssociation(instrumentname, panelname)[source]
Sets the panel name associated to an instrument
- setModifiableByUser(modifiable)[source]
Reimplemented to acept/reject drops based on whether the widget is modifiable by the user. See
TaurusBaseComponent.setModifiableByUser()
- showSDMInfo()[source]
pops up a dialog showing the current information from the Shared Data Manager
- tabifyArea(area)[source]
tabifies all panels in a given area.
- Parameters:
area (Qt.DockWidgetArea)
Warning
This method is deprecated