TaurusForm
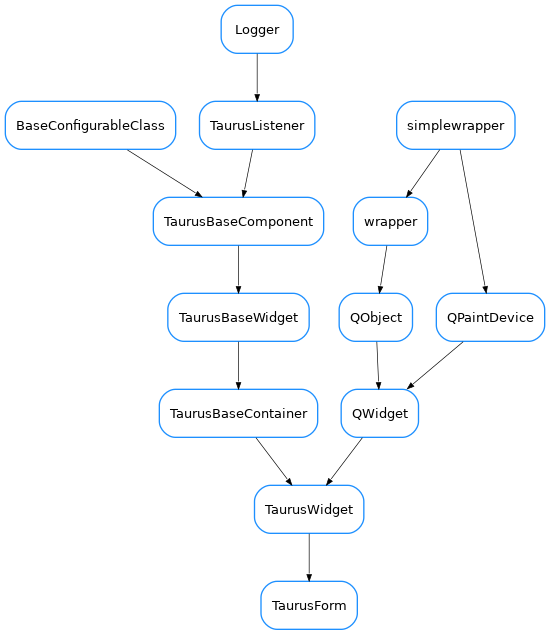
- class TaurusForm(parent=None, formWidget=None, buttons=None, withButtons=True, designMode=False)[source]
A form containing specific widgets for interacting with a given list of taurus attributes and/or devices.
Its model is a list of attribute and/or device names to be shown. Each item is represented in a row consisting of a label, a read widget, a write widget, a units widget and an “extra” widget (some of them may not be shown) which are vertically aligned with their counterparts from other items.
By default a
TaurusValue
object is used for each item, but this can be changed and customizations can be provided by enabling/disabling item factories withsetItemFactories()
.Item objects can be accessed by index using a list-like notation:
form = TaurusForm() form.model = ['sys/tg_test/1/'+a for a in ('short_image','float_scalar')] form[0].labelConfig = 'dev_alias' form[-1].writeWidgetClass = 'TaurusWheelEdit' print(len(form)) # --> outputs '2' (the length of the form is the number of items)
By default, the form provides global Apply and Cancel buttons.
You can also see some code that exemplifies the use of TaurusForm in Taurus coding examples
Import from
taurus.qt.qtgui.panel
as:from taurus.qt.qtgui.panel import TaurusForm
- addModels(modelNames)[source]
Adds models to the existing ones:
- Parameters:
modelNames (sequence<str>) – the names of the models to be added
See also
- compact
- getCustomWidgetMap(**kwargs)
Returns the map used to create custom widgets.
- return:
a dictionary whose keys are device type strings (i.e. see
PyTango.DeviceInfo
) and whose values are tuples of classname,args,kwargs- rtype:
dict<str,tuple>
Deprecated since version 4.6.5: Use item factories instead
- getFormWidget(**kwargs)
- Returns a tuple that can be used for creating a widget for a given
model.
- param model:
a taurus model name for which the new item of the form will be created
- type model:
str
- return:
a tuple containing a class, a list of args and a dict of keyword args. The args and the keyword args can be passed to the class constructor
- rtype:
tuple<type,list,dict>
Deprecated since version 4.6.5: Use item factories instead
- getItemByModel(model, index=0)[source]
returns the child item with given model. If there is more than one item with the same model, the index parameter can be used to distinguish among them Please note that his index is only relative to same-model items!
- getItemFactories(return_disabled=False)[source]
returns the list of item factories entry points currently in use
- Parameters:
return_disabled – If False (default), it returns only a list of the enabled factories. If True, it returns a tuple containing two lists: the enabled and the available but disabled factories.
- classmethod getQtDesignerPluginInfo()[source]
Returns pertinent information in order to be able to build a valid QtDesigner widget plugin.
The dictionary returned by this method should contain at least the following keys and values:
‘module’ : a string representing the full python module name (ex.: ‘taurus.qt.qtgui.base’)
‘icon’ : a string representing valid resource icon (ex.: ‘designer:combobox.png’)
- ‘container’a bool telling if this widget is a container widget or
not.
This default implementation returns the following dictionary:
{ 'group' : 'Taurus [Unclassified]', 'icon' : 'logos:taurus.png', 'container' : False }
- Returns:
a map with pertinent designer information
- Return type:
- model
- modifiableByUser
whether the user can change the contents of the widget
- Returns:
True if the user is allowed to modify the look&feel
- Return type:
- parentModelChanged(parentmodel_name)[source]
Invoked when the Taurus parent model changes
- Parameters:
parentmodel_name (str) – the new name of the parent model
- removeModels(modelNames)[source]
Removes models from those already in the form.
- Parameters:
modelNames (sequence<str>) – the names of the models to be removed
See also
- resetFormWidget(**kwargs)
Deprecated since version 4.6.5: Use item factories instead
- resetModel(**kwargs)[source]
Sets the model name to the empty string
- Parameters:
key (object) – the model key. Defaults to first element of .modelKeys
- setCustomWidgetMap(**kwargs)
Sets a map map for custom widgets.
- param cwmap:
a dictionary whose keys are device type strings (i.e. see
PyTango.DeviceInfo
) and whose values are tuples of classname,args,kwargs- type cwmap:
dict<str,tuple>
Deprecated since version 4.6.5: Use item factories instead
- setFormWidget(**kwargs)
Deprecated since version 4.6.5: Use item factories instead
- setItemFactories(include=None, exclude=None)[source]
Selects and prioritizes the factories to be used to create the form’s items.
TaurusForm item factories are functions that receive a TaurusModel as their only argument and return either a TaurusValue-like instance or None in case the factory does not handle the given model.
The factories are selected using their entry point names as registered in the “taurus.form.item_factories” entry point group.
The factories entry point name is up to the registrar of the entry point (typically a taurus plugin) and should be documented by the registrar to allow for selection and prioritization.
The selection and prioritization is done using
taurus.core.util.plugin.selectEntryPoints()
. See it for more details.The selected list is updated in the form, and returned.
The default values for the include and exclude arguments are defined in tauruscustomsettings.T_FORM_ITEM_FACTORIES
- Parameters:
include (tuple) – The members in the tuple can be: Regexp patterns (in string or compiled form) matching the names to be included in the selection. They can also be item factory functions (which then are wrapped in an EntryPoint-like object and included in the selection).
exclude (tuple) – Regexp patterns ( either str or
re.Pattern
objects) matching registered names to be excluded.
- Returns:
selected item factories entry points
- Return type:
- setModifiableByUser(modifiable)[source]
sets whether the user can change the contents of the form (e.g., via Modify Contents in the context menu) Reimplemented from
TaurusWidget.setModifiableByUser()
- Parameters:
modifiable (bool)
See also
TaurusWidget.setModifiableByUser()
- useParentModel
(deprecated))
- withButtons