TaurusFactory
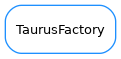
- class TaurusFactory[source]
The base class for valid Factories in Taurus.
Import from
taurus.core.taurusfactory
as:from taurus.core.taurusfactory import TaurusFactory
- DefaultPollingPeriod = 3000
- addAttributeToPolling(attribute, period, unsubscribe_evts=False)[source]
Activates the polling (client side) for the given attribute with the given period (seconds).
- Parameters:
attribute (taurus.core.taurusattribute.TaurusAttribute) – the attribute to be added
period (float) – polling period (in seconds)
unsubscribe_evts (bool) – whether or not to unsubscribe from events
- caseSensitive = True
- cleanUp()[source]
Reimplement if you need to execute code on program execution exit. Default implementation does nothing.
- elementTypesMap = None
- findObjectClass(absolute_name)[source]
Obtain the class object corresponding to the given name.
Note, this generic implementation expects that derived classes provide a an attribute called elementTypesMap consisting in a dictionary whose keys are TaurusElementTypes and whose values are the corresponding specific object classes. e.g., the FooFactory should provide:
class FooFactory(TaurusFactory): elementTypesMap = {TaurusElementType.Authority: FooAuthority, TaurusElementType.Device: FooDevice, TaurusElementType.Attribute: FooAttribute, } (...)
- Parameters:
absolute_name (str) – the object absolute name string
- Returns:
a TaurusModel class derived type or None if the name is not valid
- Return type:
- getAttribute(name)[source]
Obtain the model object corresponding to the given attribute name. If the corresponding attribute already exists, the existing instance is returned. Otherwise a new instance is stored and returned.
- Parameters:
name (str) – attribute name
- Returns:
a taurus.core.taurusattribute.TaurusAttribute object
- Raises:
- TaurusException:
if the given name is invalid.
- getAuthority(name=None)[source]
Obtain the model object corresponding to the given authority name. If the corresponding authority already exists, the existing instance is returned. Otherwise a new instance is stored and returned.
- Parameters:
name (str) – authority name
- Returns:
a taurus.core.taurusauthority.TaurusAuthority object
- Raises:
- TaurusException:
if the given name is invalid.
- getDevice(name, **kw)[source]
Obtain the model object corresponding to the given device name. If the corresponding device already exists, the existing instance is returned. Otherwise a new instance is stored and returned.
- Parameters:
name (str) – device name
- Returns:
a taurus.core.taurusdevice.TaurusDevice object
- Raises:
- TaurusException:
if the given name is invalid.
- getSerializationMode()[source]
Gives the serialization operation mode.
- Returns:
the current serialization mode
- Return type:
TaurusSerializationMode
- getValidTypesForName(name, strict=None)[source]
Returns a list of all Taurus element types for which name is a valid model name (while in many cases a name may only be valid for one element type, this is not necessarily true in general)
In this base implementation, name is checked first for Attribute, then for Device and finally for Authority, and the return value is sorted in that same order.
If a given schema requires a different ordering, reimplement this method
- Parameters:
name (str) – taurus model name
- Returns:
where element can be one of: Attribute, Device or Authority
- Return type:
list<TaurusElementType.element>
- getValidatorFromName(name)[source]
Obtain the validator object corresponding to the given model name. If the model name is not valid for any TaurusModel class, it returns None
- isPollingEnabled()[source]
Tells if the Taurus polling is enabled
- Returns:
whether or not the polling is enabled
- Return type:
- removeAttributeFromPolling(attribute)[source]
Deactivate the polling (client side) for the given attribute. If the polling of the attribute was not previously enabled, nothing happens.
- Parameters:
attribute (taurus.core.taurusattribute.TaurusAttribute) – the attribute to be removed
- schemes = ()
- setSerializationMode(mode)[source]
Sets the serialization mode for the system.
- Parameters:
mode (TaurusSerializationMode) – the new serialization mode