BZ2Codec
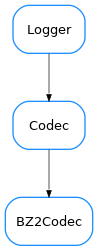
- class BZ2Codec[source]
A codec able to encode/decode to/from BZ2 format. It uses the
bz2
moduleExample:
>>> from taurus.core.util.codecs import CodecFactory >>> # first encode something >>> data = 100 * "Hello world\n" >>> cf = CodecFactory() >>> codec = cf.getCodec('bz2') >>> format, encoded_data = codec.encode(("", data)) >>> print(len(data), len(encoded_data)) 1200, 68 >>> format, decoded_data = codec.decode((format, encoded_data)) >>> print(decoded_data[20]) 'Hello world\nHello wo'
Import from
taurus.core.util.codecs
as:from taurus.core.util.codecs import BZ2Codec