Utf8Codec
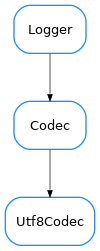
- class Utf8Codec[source]
A codec able to encode/decode utf8 strings to/from bytes. Useful to adapt i/o encodings in a codec pipe.
Example:
>>> from taurus.core.util.codecs import CodecFactory >>> cf = CodecFactory() >>> codec = cf.getCodec('zip_utf8_json') >>> >>> # first encode something >>> data = { 'hello' : 'world', 'goodbye' : 1000 } >>> format, encoded_data = codec.encode(("", data)) >>> >>> # now decode it >>> _, decoded_data = codec.decode((format, encoded_data)) >>> print(decoded_data)
Import from
taurus.core.util.codecs
as:from taurus.core.util.codecs import Utf8Codec