ThreadDict
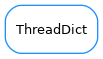
- class ThreadDict(other=None, read_method=None, write_method=None, timewait=0.1, threaded=True)[source]
Thread safe dictionary with redefinable read/write methods and a background thread for hardware update. All methods are thread-safe using @self_lock decorator.
Note
any method decorated in this way CANNOT call other decorated methods! All values of the dictionary will be automatically updated in a separate Thread using read_method provided. Any value overwritten in the dict should launch the write_method.
Briefing:
a[2] equals to a[2]=read_method(2) a[2]=1 equals to a[2]=write_method(2,1)
Import from
taurus.core.util.containers
as:from taurus.core.util.containers import ThreadDict
- copy() a shallow copy of D
- items() a set-like object providing a view on D's items
- keys() a set-like object providing a view on D's keys
- pop(k[, d]) v, remove specified key and return the corresponding value.
If key is not found, default is returned if given, otherwise KeyError is raised
- update([E, ]**F) None. Update D from dict/iterable E and F.
If E is present and has a .keys() method, then does: for k in E: D[k] = E[k] If E is present and lacks a .keys() method, then does: for k, v in E: D[k] = v In either case, this is followed by: for k in F: D[k] = F[k]
- values() an object providing a view on D's values