BaseConfigurableClass
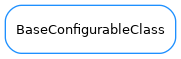
- class BaseConfigurableClass(**kwargs)[source]
A base class defining the API for configurable objects.
Note
One implicit requisite is that a configurable object must also provide a meth:`objectName method which returns the object name. This is typically fulfilled by inheriting from QObject.
Using objects that inherit from
BaseConfigurableClass
automates saving and restoring of application settings and also enables the use of perspectives in Taurus GUIs.The basic idea is that each object/widget in your application is responsible for providing a dictionary containing information on its properties (see
createConfig()
). The same object/widget is also responsible for restoring such properties when provided with a configuration dictionary (seeapplyConfig()
).For a certain property to be saved/restored it is usually enough to register it using
registerConfigProperty()
. When the objects are structured in a hierarchical way (e.g. as the widgets in a Qt application), the parent widget can (should) delegate the save/restore of its children to the children themselves. This delegation is done by registering the children usingregisterConfigDelegate()
.Consider the following example: I am creating a groupbox container which contains a
TaurusForm
and I want to save/restore the state of the checkbox and the properties of the form:# The class looks like this: class MyBox(Qt.QGroupBox, BaseConfigurableClass): def __init__(self): ... self.form = TaurusForm() ... self.registerConfigProperty( self.isChecked, self.setChecked, 'checked' ) # the TaurusForm already handles its own configuration! self.registerConfigDelegate(self.form) ... # and we can retrieve the configuration doing: b1 = MyBox() # checked is a registered property of MyBox class b1.setChecked(True) # modifiableByUser is a registered property of a TaurusForm b1.form.setModifiableByUser(True) # we get the configuration as a dictionary cfg = b1.createConfig() ... b2 = MyBox() # now b2 has the same configuration as b1 when cfg was created b2.applyConfig(cfg)
createConfig()
andapplyConfig()
methods use a dictionary for passing the configuration, butBaseConfigurableClass
also provides some other convenience methods for working with files (saveConfigFile()
andloadConfigFile()
) or as QByteArrays (createQConfig()
andapplyQConfig()
)Finally, we recommend to use
TaurusMainWindow
for all Taurus GUIs since it automates all the steps for saving properties when closing and restoring the settings on startup. It also provides a mechanism for implementing “perspectives” in your application.Import from
taurus.qt.qtcore.configuration
as:from taurus.qt.qtcore.configuration import BaseConfigurableClass
- applyConfig(configdict, depth=None)[source]
applies the settings stored in a configdict to the current object.
In most usual situations, using
registerConfigProperty()
andregisterConfigDelegate()
, should be enough to cover all needs using this method, although it can be reimplemented in children classes to support very specific configurations.- Parameters:
configdict (dict)
depth (int) – If depth = 0, applyConfig will only be called for this object, and not for any other object registered via
registerConfigurableItem()
. If depth > 0, applyConfig will be called recursively as many times as the depth value. If depth < 0 (default, see note), no limit is imposed to recursion (i.e., it will recurse for as deep as there are registered items).
Note
the default recursion depth can be tweaked in derived classes by changing the class property defaultConfigRecursionDepth
See also
- applyQConfig(qstate)[source]
restores the configuration from a qstate generated by
getQState()
.- Parameters:
qstate (QByteArray)
See also
- checkConfigVersion(configdict, showDialog=False, supportedVersions=None)[source]
Check if the version of configdict is supported. By default, the BaseConfigurableClass objects have [“__UNVERSIONED__”] as their list of supported versions, so unversioned config dicts will be accepted.
- Parameters:
configdict (dict) – configuration dictionary to check
showDialog (bool) – whether to show a QtWarning dialog if check failed (false by default)
supportedVersions (sequence<str>, or None) – supported version numbers, if None given, the versions supported by this widget will be used (i.e., those defined in self._supportedConfigVersions)
- Returns:
returns True if the configdict is of the right version
- Return type:
- createConfig(allowUnpickable=False)[source]
Returns a dictionary containing configuration information about the current state of the object.
In most usual situations, using
registerConfigProperty()
andregisterConfigDelegate()
, should be enough to cover all needs using this method, although it can be reimplemented in children classes to support very specific configurations.By default, meth:createQConfig and meth:saveConfigFile call to this method for obtaining the data.
Hint: The following code allows you to serialize the configuration dictionary as a string (which you can store as a QSetting, or as a Tango Attribute, provided that allowUnpickable==False):
import pickle s = pickle.dumps(widget.createConfig())
- Parameters:
alllowUnpickable (bool) – if False the returned dict is guaranteed to be a pickable object. This is the default and preferred option because it allows the serialization as a string that can be directly stored in a QSetting. If True, this limitation is not enforced, which allows to use more complex objects as values (but limits its persistence).
- Returns:
configurations (which can be loaded with
applyConfig()
).- Return type:
dict<str,object>
- createQConfig()[source]
returns the current configuration status encoded as a QByteArray. This state can therefore be easily stored using QSettings
- Returns:
(in the current implementation this is just a pickled configdict encoded as a QByteArray
- Return type:
QByteArray
See also
restoreQConfig()
- defaultConfigRecursionDepth = -1
- getConfigurableItemNames()[source]
returns an ordered list of the names of currently registered configuration items (delegates and properties)
- Returns:
- Return type:
list<unicode>
- loadConfigFile(ifile=None)[source]
Reads a file stored by
saveConfig()
and applies the settings- Parameters:
ifile (file or string) – file or filename from where to read the configuration
- Returns:
file name used
- Return type:
- registerConfigDelegate(delegate, name=None)[source]
Registers the given object as a delegate for configuration. Delegates are typically other objects inheriting from BaseConfigurableClass (or at least they must provide the following methods:
createConfig (as provided by, e.g., BaseConfigurableClass)
applyConfig (as provided by, e.g., BaseConfigurableClass)
objectName (as provided by, e.g., QObject)
- Parameters:
delegate (BaseConfigurableClass) – The delegate object to be registered.
name (str) – The name to be used as a key for this item in the configuration dictionary. If None given, the object name is used by default.
Note
the registration order will be used when restoring configurations
- registerConfigProperty(fget, fset, name)[source]
Registers a certain property to be included in the config dictionary.
In this context a “property” is some named value that can be obtained via a getter method and can be set via a setter method.
- Parameters:
fget (method or str) – method (or name of a method) that gets no arguments and returns the value of a property.
fset (method or str) – method (or name of a method) that gets as an argument the value of a property, and sets it
name (str) – The name to be used as a key for this property in the configuration dictionary
Note
the registration order will be used when restoring configurations
- resetConfigurableItems()[source]
clears the record of configurable items depending of this object
See also
- saveConfigFile(ofile=None)[source]
Stores the current configuration on a file
- Parameters:
ofile (file or string) – file or filename to store the configuration
- Returns:
file name used
- Return type: