QTaurusBaseListener
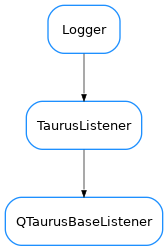
- class QTaurusBaseListener(name=None, parent=None)[source]
Base class for QObjects listening to taurus events.
Note
getSignaller()
is now unused and deprecated. This is because taurusEvent is implemented usingbaseSignal()
, that doesn’t require the class to inherit from QObject.Import from
taurus.qt.qtcore.taurusqlistener
as:from taurus.qt.qtcore.taurusqlistener import QTaurusBaseListener
- eventReceived(evt_src, evt_type, evt_value)[source]
The basic implementation of the event handling chain is as follows:
eventReceived just calls
fireEvent()
which emits a “taurusEvent” PyQt signal that is connected (bypreAttach()
) to thefilterEvent()
method.After filtering,
handleEvent()
is invoked with the resulting filtered event
Note
in the earlier steps of the chain (i.e., in
eventReceived()
/fireEvent()
), the code is executed in a Python thread, while from eventFilter ahead, the code is executed in a Qt thread. When writing widgets, one should normally work on the Qt thread (i.e. reimplementinghandleEvent()
)
- filterEvent(evt_src=-1, evt_type=-1, evt_value=-1)[source]
The event is processed by each and all filters in strict order unless one of them returns None (in which case the event is discarded)
- fireEvent(evt_src=None, evt_type=None, evt_value=None)[source]
Emits a “taurusEvent” signal.
It is unlikely that you may need to reimplement this method in subclasses. Consider reimplementing
eventReceived()
orhandleEvent()
instead depending on whether you need to execute code in the python or Qt threads, respectively
- getEventFilters()[source]
Returns the list of event filters for this widget
- Returns:
the event filters
- Return type:
sequence<callable>
- getSignaller(**kwargs)
Deprecated since version 4.0.
- handleEvent(evt_src, evt_type, evt_value)[source]
Event handling. Default implementation does nothing. Reimplement as necessary
- insertEventFilter(filter, index=-1)[source]
insert a filter in a given position
- Parameters:
filter (callable(evt_src, evt_type, evt_value)) – a filter
index (int) – index to place the filter (default = -1 meaning place at the end)
See also: setEventFilters
- setEventFilters(filters=None)[source]
sets the taurus event filters list.
The filters are run in order, using each output to feed the next filter. A filter must be a function that accepts 3 arguments
(evt_src, evt_type, evt_value)
If the event is to be ignored, the filter must return None. If the event is not to be ignored, filter must return a(evt_src, evt_type, evt_value)
tuple which may (or not) differ from the input.For a library of common filters, see taurus/core/util/eventfilters.py
- Parameters:
filters (sequence) – a sequence of filters
See also: insertEventFilter
- property taurusEvent
Base signal taurusEvent