TaurusInputPanel
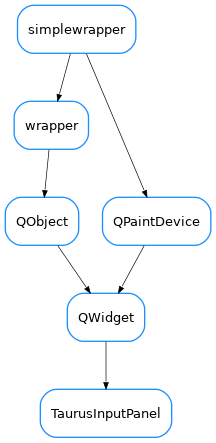
- class TaurusInputPanel(input_data, parent=None)[source]
A panel design to get an input from the user.
The input_data is a dictionary which contains information on how to build the input dialog. It must contains the following keys:
prompt <str>: message to be displayed
The following are optional keys (and their corresponding default values):
title <str> (doesn’t have default value)
key <str> (doesn’t have default value): a label to be presented left to the input box represeting the label
unit <str> (doesn’t have default value): a label to be presented right to the input box representing the units
data_type <str or sequence> (‘String’): type of data to be requested. Standard accepted data types are ‘String’, ‘Integer’, ‘Float’, ‘Boolean’, ‘Text’. A list of elements will be interpreted as a selection. Default TaurusInputPanel class will interpret any custom data types as ‘String’ and will display input widget accordingly. Custom data types can be handled differently by supplying a different input_panel_klass.
minimum <int/float>: minimum value (makes sense when data_type is ‘Integer’ or ‘Float’)
maximum <int/float>: maximum value (makes sense when data_type is ‘Integer’ or ‘Float’)
step <int/float> (1): step size value (makes sense when data_type is ‘Integer’ or ‘Float’)
decimals <int> (1): number of decimal places to show (makes sense when data_type is ‘Float’)
default_value <obj> (doesn’t have default value): default value
allow_multiple <bool> (False): allow more than one value to be selected (makes sense when data_type is a sequence of possibilities)
Example:
app = Qt.QApplication([]) class Listener(object): def on_accept(self): print("user selected", self.panel.value()) d = dict( prompt="What's your favourite car brand?", data_type=["Mazda", "Skoda", "Citroen", "Mercedes", "Audi"], default_value="Mercedes" ) w = TaurusInputPanel(d) l = Listener() l.panel = w w.connect(w.buttonBox(), Qt.SIGNAL("accepted()"), l.on_accept) w.show() app.exec_()
Import from
taurus.qt.qtgui.panel
as:from taurus.qt.qtgui.panel import TaurusInputPanel
- addButton(button, role=3)[source]
Adds the given button with the given to the button box
- Parameters:
button (PyQt5.Qt.QPushButton) – the button to be added
role (PyQt5.Qt.QDialogButtonBox.ButtonRole) – button role
- buttonBox()[source]
Returns the button box from this panel
- Returns:
the button box from this panel
- Return type:
PyQt5.Qt.QDialogButtonBox
- getText()[source]
Returns the current text of this panel
- Returns:
the text for this panel
- Return type:
- loadUi(filename=None, path=None)