AttributeEventWait
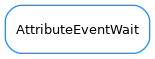
- class AttributeEventWait(attr=None)[source]
Class designed to connect to a
taurus.core.taurusattribute.TaurusAttribute
and fire events or wait for a certain event.Import from
taurus.core.util.event
as:from taurus.core.util.event import AttributeEventWait
- connect(attr)[source]
Connect to the given attribute
- Parameters:
attr (taurus.core.taurusattribute.TaurusAttribute) – the attribute to connect to
- eventReceived(s, t, v)[source]
Event listener method for the underlying attribute. Do not call this method. It will be called internally when the attribute generates an event.
- fireEvent(v)[source]
Notifies that a given event has arrived This function is protected inside with the object’s lock. Do NOT call this function when you have the lock acquired on this object.
- Parameters:
v (object) – event value
- getLastRecordedEvent()[source]
returns the value of the last recorded event or None if no event has been received or the last event was an error event
- Returns:
the last event value to be recorded
- Return type:
- getRecordedEvent(v)[source]
Returns the the recorded local timestamp for the event with the given value or None if no event with the given value has been recorded.
- getRecordedEvents()[source]
Returns a reference to the internal dictionary used to store the internal events. Modify the return dictionary at your own risk!
- Returns:
reference to the internal event dictionary
- Return type:
- waitEvent(val, after=0, equal=True, timeout=None, retries=-1, any=False)[source]
Wait for an event with the given value.
- Parameters:
val (object) – value to compare
after (float) – timestamp. wait for events comming after the given time. default value is 0 meaning any event after Jan 1, 1970
equal (bool) – compare for equality. equal=True means an event with the given value, equal=False means any event which as a different value
timeout (float) – maximum time to wait (seconds). Default is None meaning wait forever.
retries – number of maximum retries of max timeout to attempts. Default is -1 meaning infinite number of retries. 0 means no wait. Positive number is obvious.
any (bool) – if any is True ignore ‘val’ parameter and accept any event. If False (default),check with given ‘val’ parameter
- waitForEvent(values, after=0, equal=True, timeout=None, reactivity=None, ignore_values=False)[source]
Wait for an event that matches some given conditions.
- Parameters:
values (seq<object>) – values to compare with the received event value
after (float) – timestamp. wait for events comming after the given time. default value is 0 meaning any event after Jan 1, 1970
equal (bool) – determines how the comparison is made. If True, the wait will finish when the event matches any of the given values. If False, the wait will finish when the event differs from all the given values.
timeout (float) – maximum time to wait (seconds). Default is None meaning wait forever.
reactivity – setting reactivity (seconds) waranties reactions to aborts comming from other threads in at most the reactivity time. Default is None meaning no reactivity within timeout.
ignore_values (bool) – if any is True ignore ‘values’ parameter and accept any event. If False (default),check with given ‘values’ parameter
- Returns:
The return value is True unless a given timeout expired, in which case it is False.
- Return type: