EventListener
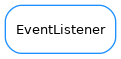
- class EventListener[source]
A class that listens for an event with a specific value
Note: Since this class stores for each event value the last timestamp when it occured, it should only be used for events for which the event value domain (possible values) is limited and well known (ex: an enum)
Import from
taurus.core.util.event
as:from taurus.core.util.event import EventListener
- fireEvent(v)[source]
Notifies that a given event has arrived This function is protected inside with the object’s lock. Do NOT call this function when you have the lock acquired on this object.
- Parameters:
v (object) – event value
- waitEvent(val, after=0, equal=True)[source]
Wait for an event with the given value. You MUST protect this function with this object’s lock before calling this method and always unlock it afterward, of course:
from taurus.core.util.event import EventListener class MyEvtListener(EventListener): # Your specific listener code here pass evt_listener = EventListener() try: evt_listener.lock() t = time.time() go() evt_listener.waitEvent(Stop, t) finally: evt_listener.unlock()
- Parameters: