TaurusBaseWidget
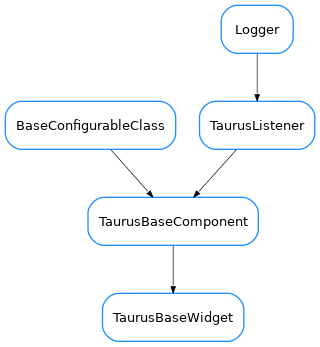
- class TaurusBaseWidget(name='', parent=None, designMode=False, **kwargs)[source]
The base class for all Qt Taurus widgets.
Note
Any class which inherits from TaurusBaseWidget is expected to also inherit from QWidget (or from a QWidget derived class)
Import from
taurus.qt.qtgui.base
as:from taurus.qt.qtgui.base import TaurusBaseWidget
- changeEvent(evt)[source]
overwrites QWidget.changeEvent(self, evt) to handle the ParentChangeEvent in case this widget is using the parent model. Always calls the QWidget.changeEvent in order not to lose events
- emitValueChanged(*args)[source]
Connect the specific XXXXChanged signals from derived classes to this method in order to have a unified signal which can be used by Taurus Widgets
- getAutoTooltip()[source]
Returns if the widget is automatically generating a tooltip based on the current widget model.
- Returns:
True if automatically generating tooltip or False otherwise
- Return type:
- getDropEventCallback()[source]
returns the method to be called when a dropping event occurs. The default implementation returns self.setModel. Reimplement it subclasses to call different methods.
- Returns:
method to be called when a dropping event occurs.
- Return type:
callable
- getModelMimeData()[source]
Returns a MimeData object containing the model data. The default implementation fills the TAURUS_MODEL_MIME_TYPE. If the widget’s Model class is Attribute or Device, it also fills TAURUS_ATTR_MIME_TYPE or TAURUS_DEV_MIME_TYPE, respectively
- Returns:
MimeData object containing the model data.
- Return type:
QMimeData
- getParentTaurusComponent(**kwargs)
Deprecated since version 4.3.2.
- getQtClass(bases=None)[source]
Returns the parent Qt class for this widget
- Parameters:
bases (sequence<class> or None) – the list of class objects. If None is given (default) it uses the object base classes from __bases__
- Returns:
the QWidget class object
- Return type:
QWidget class
- classmethod getQtDesignerPluginInfo()[source]
Returns pertinent information in order to be able to build a valid QtDesigner widget plugin.
The dictionary returned by this method should contain at least the following keys and values:
‘module’ : a string representing the full python module name (ex.: ‘taurus.qt.qtgui.base’)
‘icon’ : a string representing valid resource icon (ex.: ‘designer:combobox.png’)
- ‘container’a bool telling if this widget is a container widget or
not.
This default implementation returns the following dictionary:
{ 'group' : 'Taurus [Unclassified]', 'icon' : 'logos:taurus.png', 'container' : False }
- Returns:
a map with pertinent designer information
- Return type:
- getSupportedMimeTypes()[source]
returns a list of supported mimeTypes that this widget support (ordered by priority). If none is set explicitely via
setSupportedMimeTypes()
, a best effort will be tried based on the model class..seealso:
setSupportedMimeTypes()
This provides only a very basic implementation. Reimplement in derived classes if needed
- Returns:
list of MIME type names
- Return type:
list<str>
- handleEvent(evt_src, evt_type, evt_value)[source]
very basic and generalistic handling of events.
Override when necessary.
- handleMimeData(mimeData, method)[source]
Selects the most appropriate data from the given mimeData object (in the order returned by
getSupportedMimeTypes()
) and passes it to the given method.- Parameters:
mimeData (QMimeData) – the MIME data object from which the model is to be extracted
method (callable<str>) – a method that accepts a string as argument. This method will be called with the data from the mimeData object
- Returns:
returns the MimeType used if the model was successfully set, or None if the model could not be set
- Return type:
str or None
- isDragEnabled()[source]
whether the user can drag data from this widget
- Returns:
True if the user can drag data
- Return type:
- property modelChanged
Base signal modelChanged
- mousePressEvent(event)[source]
reimplemented to record the start position for drag events. See
QWidget
- onSetFormatter()[source]
Slot to allow interactive setting of the Formatter.
See also
TaurusBaseWidget.__showFormatterDlg()
,TaurusBaseComponent.displayValue()
,tauruscustomsettings.DEFAULT_FORMATTER
- parentModelChanged(parentmodel_name)[source]
Invoked when the Taurus parent model changes
- Parameters:
parentmodel_name (str) – the new name of the parent model
- recheckTaurusParent()[source]
Forces the widget to recheck its Taurus parent. Taurus Widgets will in most situations keep track of changes in their taurus parenting, but in some special cases (which unfortunately tend to occur when using Qt Designer) they may not update it correctly.
If this happens, you can manually call this method.
For more information, check the
issue demo example
- safeApplyOperations(ops=None)[source]
Applies the given operations (or the pending operations if None passed)
- Parameters:
ops (sequence<taurus.core.taurusoperation.TaurusOperation> or None) – list of operations to apply. If None is given (default) the component fetches the pending operations
- Returns:
False if the apply was aborted by the user or if the widget is in design mode. True otherwise.
- Return type:
- setAutoTooltip(yesno)[source]
Determines if the widget should automatically generate a tooltip based on the current widget model.
- Parameters:
yesno (bool) – True to automatically generate tooltip or False otherwise
- setDisconnectOnHide(disconnect)[source]
Sets/unsets disconnection on hide event
- Parameters:
disconnect (bool) – whether or not to disconnect on hide event
- setDragEnabled(enabled)[source]
sets whether the user is allowed to drag data from this widget
- Parameters:
enabled (bool) – if True, dragging is enabled. False disables
- setModelInConfig(yesno)[source]
extends
TaurusBaseComponent.setModelInConfig()
to include also the “useParentModel” property
- setModifiableByUser(modifiable)[source]
Reimplemented to acept/reject drops based on whether the widget is modifiable by the user. See
TaurusBaseComponent.setModifiableByUser()
- setSupportedMimeTypes(mimetypes)[source]
sets the mimeTypes that this widget support
- Parameters:
mimetypes (list<str>) – list (ordered by priority) of MIME type names
- setUseParentModel(yesno)[source]
..note:: Deprecated since v4.3.2
Sets/unsets using the parent model.
Note
in some cases you may need to call
recheckTaurusParent()
after reparenting of some of this widget’s ancestors- Parameters:
yesno (bool) – whether or not to use parent model
See also
- showFormatterDlg(**kwargs)
- deprecated because it does not distinguish between a user cancelling
the dialog and the user selecting None as a formatter.
Deprecated since version 4.6.2: Use onSetFormatter instead
- updatePendingOpsStyle()[source]
This method should be reimplemented by derived classes that want to change their appearance depending whether there are pending operations or not
- updateStyle()[source]
Updates the widget style. Default implementation just calls QWidget.update()
Override when necessary.
- property valueChangedSignal
Base signal valueChanged