TaurusBaseComponent
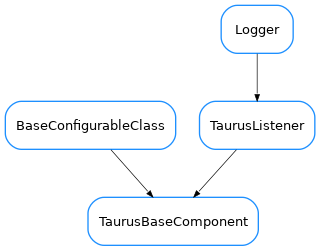
- class TaurusBaseComponent(name='', parent=None, designMode=False, **kwargs)[source]
A generic Taurus component.
Note
Any class which inherits from TaurusBaseComponent is expected to also inherit from QObject (or from a QObject derived class).
Note
getSignaller()
is now unused and deprecated. This is because taurusEvent is implemented usingbaseSignal()
, that doesn’t require the class to inherit from QObject.Import from
taurus.qt.qtgui.base
as:from taurus.qt.qtgui.base import TaurusBaseComponent
- FORMAT = None
- MLIST = 0
MLIST is a constant that can be passed as the value of the key kwarg in the multi-model API to indicate that the class should act as a model-container regarding to this key (e.g. TaurusBaseComponent.setModel(m, key=MLIST) will treat m as a list of models.
- applyPendingOperations(ops=None)[source]
Applies operations without caring about danger messages. Use
TaurusBaseWidget.safeApplyOperation()
if you want to warn the user before applying- Parameters:
ops (sequence<taurus.core.taurusoperation.TaurusOperation> or None) – list of operations to apply. If None is given (default) the component fetches the pending operations
- contextMenuEvent(event)[source]
DEPRECATED: Until v4.5.3a, the default implementation of contextMenuEvent showed the content of taurusMenu as a context menu. But this resulted in unwanted behaviour when the widget already implemented its own context menu (see https://gitlab.com/taurus-org/taurus/-/issues/905 )
Therefore this feature was disabled in 4.5.3a.
If you still want to show the contents of taurusMenu as a context menu, you can explicitly reimplement the contextMenuEvent method as:
def contextMenuEvent(self, event): self.taurusMenu.exec_(event.globalPos())
- defaultFormatDict = {<class 'float'>: '{:.{bc.modelObj.precision}f}', <class 'pint.Quantity'>: '{:~.{bc.modelObj.precision}f}', <enum 'Enum'>: '{0.name}'}
- deleteLater()[source]
Reimplements the Qt.QObject deleteLater method to ensure that the this object stops listening its model.
- displayValue(v)[source]
Returns a string representation of the given value
This method will use a format string which is determined dynamically from
FORMAT
.By default TaurusBaseComponent.FORMAT is set to
defaultFormatter()
, which makes use ofdefaultFormatDict
.In order to customize the formatting behaviour, one can use
setFormat()
to alter the formatter of an specific instance (recommended) or changedefaultFormatDict
orFORMAT
directly at class level.The formatter can be set to a python format string [1] or a callable that returns a python format string. If a callable is used, it will be called with the following keyword arguments:
dtype: the data type of the value to be formatted
basecomponent: the affected widget
The following are some examples for customizing the formatting:
- Change the format for widget instance `foo`::
foo.setFormat(“{:.2e}”)
Change FORMAT for all widgets (using a string):
TaurusBaseComponent.FORMAT = "{:.2e}"
Change FORMAT for all TaurusLabels (using a callable):
def baseFormatter(dtype=None, basecomponent=None, **kwargs): return "{:.1f}" TaurusLabel.FORMAT = baseFormatter
Use the defaultFormatDict but modify the format string for dtype=str:
TaurusLabel.defaultFormatDict.update({"str": "{!r}"})
See also
tauruscustomsettings.DEFAULT_FORMATTER
, –default-formatter option inTaurusApplication
,TaurusBaseWidget.onSetFormatter()
- eventReceived(evt_src, evt_type, evt_value)[source]
The basic implementation of the event handling chain is as follows:
eventReceived just calls
fireEvent()
which emits a “taurusEvent” PyQt signal that is connected (bypreAttach()
) to thefilterEvent()
method.After filtering,
handleEvent()
is invoked with the resulting filtered event
Note
in the earlier steps of the chain (i.e., in
eventReceived()
/fireEvent()
), the code is executed in a Python thread, while from eventFilter ahead, the code is executed in a Qt thread. When writing widgets, one should normally work on the Qt thread (i.e. reimplementinghandleEvent()
)
- filterEvent(evt_src=-1, evt_type=-1, evt_value=-1)[source]
The event is processed by each and all filters in strict order unless one of them returns None (in which case the event is discarded)
- findModelClass(**kwargs)
Deprecated since version 5.1.0: Use getModelClass instead
- fireBufferedEvents()[source]
Fire all events currently buffered (and flush the buffer)
- Note: this method is normally called from an event buffer timer thread
but it can also be called any time the buffer needs to be flushed
- fireEvent(evt_src=None, evt_type=None, evt_value=None)[source]
Emits a “taurusEvent” signal. It is unlikely that you need to reimplement this method in subclasses. Consider reimplementing
eventReceived()
orhandleEvent()
instead depending on whether you need to execute code in the python or Qt threads, respectively
- getDangerMessage()[source]
Returns the current apply danger message or None if the apply operation is safe
- Returns:
the apply danger message
- Return type:
str or None
- getDisplayValue(cache=True, fragmentName=None, *, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Returns a string representation of the model value associated with this component.
- Parameters:
cache (bool) – (ignored, just for bck-compat).
fragmentName (str or None) – the returned value will correspond to the given fragmentName. If None passed, self.modelFragmentName will be used, and if None is set, the defaultFragmentName of the model will be used instead.
key (object) – the model key. Defaults to first element of .modelKeys
- Returns:
a string representation of the model value.
- Return type:
- getEventBufferPeriod()[source]
Returns the event buffer period
- Returns:
period (in s). 0 means event buffering is disabled.
- Return type:
- getEventFilters(preqt=False)[source]
Returns the list of event filters for this widget
- Parameters:
preqt (bool) – If true, return the pre-filters (that are applied in eventReceived, at the python thread), otherwise, return the filters to be applied at the main Qt thread (default)
- Returns:
the event filters
- Return type:
sequence<callable>
- getForceDangerousOperations()[source]
Returns if apply dangerous operations is forced
- Returns:
wheter or not apply dangerous operations is forced
- Return type:
- getFormat()[source]
Method to get the FORMAT attribute for this instance.
- Returns:
a string of the current format. It could be a python format string or a callable string representation.
- Return type:
- getFormatedToolTip(cache=True)[source]
Returns a string with contents to be displayed in a tooltip.
- getFullModelName(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Returns the full name of the model object for the given key.
- getModel(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Returns the model name for this component.
- getModelClass(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Return the class object for the widget. Default behavior is to do a ‘best effort’ to determine which model type corresponds to the current model name. Overwrite as necessary.
- Parameters:
key (object) – the model key. Defaults to first element of .modelKeys
- Returns:
The class object corresponding to the type of Taurus model this widget handles or None if no valid class is found.
- Return type:
class TaurusModel or None
- getModelFragmentObj(fragmentName=None, *, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Returns a fragment object of the model. A fragment of a model is a python attribute of the model object.
Fragment names including dots will be used to recursively get fragments of fragments.
For a simple fragmentName (no dots), this is roughly equivalent to getattr(self.getModelObj(), fragmentName)
If the model does not have that fragment,
AttributeError
is raised (other exceptions may be raised when accessing the fragment as well)- Parameters:
- Returns:
the member of the modelObj referred by the fragment.
- Return type:
obj
- getModelIndexValue()[source]
Called inside getDisplayValue to use with spectrum attributes. By default not used, but some widget might want to support this feature.
Note
getModelIndexValue() is only supported for the default model key
Override when needed.
- getModelName(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Returns the current model name.
- getModelNames()[source]
Returns a dict of key:modelname
- Returns:
dictionary mapping keys to model names
- Return type:
- getModelObj(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Returns the taurus model object associated to the given key or None if no taurus model is associated.
- Parameters:
key (object) – the model key. Defaults to first element of .modelKeys
- Returns:
the taurus model object
- Return type:
- getModelType(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Returns the taurus model type associated with this key or taurus.core.taurusbasetypes.TaurusElementType.Unknown if no taurus model is associated.
- Parameters:
key (object) – the model key. Defaults to first element of .modelKeys
- Returns:
the taurus model type
- Return type:
taurus.core.taurusbasetypes.TaurusElementType
- getModelValueObj(cache=True, *, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Returns the current value of the taurus model associated with this key or None if no taurus model is associated.
- Parameters:
- Returns:
the tango value object.
- Return type:
- getNoneValue()[source]
Returns the current string representation when no valid model or model value exists.
- Returns:
a string representation for an invalid value
- Return type:
- getParentModelName(**kwargs)
Deprecated since version 4.3.2.
- getParentModelObj(**kwargs)
Deprecated since version 4.3.2.
- getParentTaurusComponent(**kwargs)
Deprecated since version 4.3.2.
- getPendingOperations()[source]
Returns the sequence of pending operations
- Returns:
a list of pending operations
- Return type:
sequence<taurus.core.taurusoperation.TaurusOperation>
- getShowQuality()[source]
Returns if showing the quality as a background color
- Returns:
True if showing the quality or False otherwise
- Return type:
- getShowText()[source]
Returns if showing the display value
- Returns:
True if showing the display value or False otherwise
- Return type:
- getSignaller(**kwargs)
Deprecated since version 4.0.
- getTaurusFactory(scheme='')[source]
Returns the taurus factory singleton for the given scheme. This is just a helper method. It is the equivalent of doing:
import taurus factory = taurus.Factory(scheme)
- Parameters:
scheme (str or None) – the scheme. If scheme is an empty string, or is not passed, the scheme will be obtained from the model name. For backwards compatibility (but deprecated), passing None is equivalent to ‘tango’.
- Returns:
the TaurusFactory
- Return type:
- getTaurusManager()[source]
Returns the taurus manager singleton. This is just a helper method. It is the equivalent of doing:
import taurus manager = taurus.Manager()
- Returns:
the TaurusManager
- Return type:
- getTaurusPopupMenu(**kwargs)
Returns an xml string representing the current taurus popup menu
- return:
an xml string representing the current taurus popup menu
- rtype:
str
Deprecated since version 4.5.3a.
- getUseParentModel(**kwargs)
Deprecated since version 4.3.2.
- static get_registered_formatters()[source]
Static method to get the registered formatters :return: dict of registered formatter (keys are the registered names) :rtype: dict
- handleEvent(evt_src, evt_type, evt_value)[source]
Event handling. Default implementation does nothing. Reimplement as necessary
- hasPendingOperations()[source]
Returns if the component has pending operations
- Returns:
True if there are pending operations or False otherwise
- Return type:
- insertEventFilter(filter, index=-1, preqt=False)[source]
insert a filter in a given position
- Parameters:
filter (callable(evt_src, evt_type, evt_value) – ) a filter
index (int) – index to place the filter (default = -1 meaning place at the end)
preqt (bool) – If true, set the pre-filters (that are applied in eventReceived, at the python thread), otherwise, set the filters to be applied at the main Qt thread (default)
See also: setEventFilters
- isAttached(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Determines if this component is attached to the taurus model.
- isAutoProtectOperation()[source]
Tells if this widget’s operations are protected against exceptions
- Returns:
True if operations are protected against exceptions or False otherwise
- Return type:
- isChangeable()[source]
Tells if this component value can be changed by the user. Default implementation will return True if and only if:
this component is attached to a valid taurus model and
the taurus model is writable and
this component is not read-only
- Returns:
True if this component value can be changed by the user or False otherwise
- Return type:
- isDangerous()[source]
Returns if the apply operation for this component is dangerous
- Returns:
wheter or not the apply operation for this component is dangerous
- Return type:
- isModifiableByUser()[source]
whether the user can change the contents of the widget
- Returns:
True if the user is allowed to modify the look&feel
- Return type:
- isPaused()[source]
Return the current pause state
- Returns:
wheater or not the widget is paused
- Return type:
- isReadOnly()[source]
Determines if this component is read-only or not in the sense that the user can interact with it. Default implementation returns True.
Override when necessary.
- Returns:
whether or not this component is read-only
- Return type:
- property modelFragmentName
- modelKeys = ['']
- property modelName
- property modelObj
- postAttach(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Called inside self.attach() after actual attach is performed. Default implementation does not do anything.
Override when necessary.
- Parameters:
key (object) – the model key. Defaults to first element of .modelKeys
- postDetach(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Called inside self.detach() after actual deattach is performed. Default implementation does not do anything.
Override when necessary.
- Parameters:
key (object) – the model key. Defaults to first element of .modelKeys
- preAttach(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Called inside self.attach() before actual attach is performed. Default implementation does nothing.
Override when necessary.
- Parameters:
key (object) – the model key. Defaults to first element of .modelKeys
- preDetach(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Called inside self.detach() before actual deattach is performed. Default implementation does nothing.
Override when necessary.
- Parameters:
key (object) – the model key. Defaults to first element of .modelKeys
- resetDangerMessage()[source]
Clears the danger message. After this method is executed the apply operation for this component will be considered safe.
- resetFormat()[source]
Reset the internal format string. It forces a recalculation in the next call to
displayValue()
.
- resetModel(*, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Sets the model name to the empty string
- Parameters:
key (object) – the model key. Defaults to first element of .modelKeys
- resetModifiableByUser()[source]
Equivalent to setModifiableByUser(self.__class__._modifiableByUser)
- resetTaurusPopupMenu(**kwargs)
Resets the taurus popup menu to empty
Deprecated since version 4.5.3a.
- resetUseParentModel(**kwargs)
Deprecated since version 4.3.2.
- setAutoProtectOperation(protect)[source]
Sets/unsets this widget’s operations are protected against exceptions
- Parameters:
protect (bool) – wheater or not to protect widget operations
- setDangerMessage(dangerMessage='')[source]
Sets the danger message when applying an operation. If dangerMessage is None, the apply operation is considered safe
- Parameters:
dangerMessage (str or None) – the danger message. If None is given (default) the apply operation is considered safe
- setEventBufferPeriod(period)[source]
Set the period at wich
fireBufferedEvents()
will be called. If period is 0, the event buffering is disabled (i.e., events are fired as soon as they are received)- Parameters:
period (float) – period in seconds for the automatic event firing. period=0 will disable the event buffering.
- setEventFilters(filters=None, preqt=False)[source]
sets the taurus event filters list. The filters are run in order, using each output to feed the next filter. A filter must be a function that accepts 3 arguments
(evt_src, evt_type, evt_value)
If the event is to be ignored, the filter must return None. If the event is not to be ignored, filter must return a(evt_src, evt_type, evt_value)
tuple which may (or not) differ from the input.For a library of common filters, see taurus/core/util/eventfilters.py
- Parameters:
filters (sequence) – a sequence of filters
preqt (bool) – If true, set the pre-filters (that are applied in eventReceived, at the python thread), otherwise, set the filters to be applied at the main Qt thread (default)
Note: If you are setting a filter that applies a transformation on the parameters, you may want to generate a fake event to force the last value to be filtered as well. This can be done as in this example:
TaurusBaseComponent.fireEvent( TaurusBaseComponent.getModelObj(), taurus.core.taurusbasetypes.TaurusEventType.Periodic, TaurusBaseComponent.getModelObj().getValueObj())
See also: insertEventFilter
- setForceDangerousOperations(yesno)[source]
Forces/clears the dangerous operations
- Parameters:
yesno (bool) – force or not the dangerous operations
- setFormat(format)[source]
Method to set the FORMAT attribute for this instance. It also resets the internal format string, which will be recalculated in the next call to
displayValue()
- Parameters:
format (str or callable) – A format string or a formatter callable (or the callable name in “full.module.callable” format)
- setModel(model, *, key=<class 'taurus.qt.qtgui.base.taurusbase._DEFAULT'>)[source]
Sets/unsets the model name for the given key. If key is MLIST, a model is set for each name in the model sequence, new model keys are automatically added to the object’s
modelList
attribute and the corresponding models are attached using those keys. The new keys are of the form(MLIST, i)
wherei
is the index of the corresponding model name in the model sequence.
- setModelCheck(**kwargs)
Deprecated since version 5.1.0: Use setModel instead
- setModelInConfig(yesno)[source]
Sets whether the model-related properties should be stored for this widget when creating the config dict with
createConfig()
(and restored when callingapplyConfig()
). By default this is not enabled. The following properties are affected by this:“model”
- Parameters:
yesno (bool) – If True, the model-related properties will be registered as config properties. If False, they will be unregistered.
See also
registerConfigProperty()
,createConfig()
,applyConfig()
- setModelName(**kwargs)
Deprecated since version 5.1.0.
- setModifiableByUser(modifiable)[source]
sets whether the user is allowed to modify the look&feel
- Parameters:
modifiable (bool) – True allows the user to modify certain properties of the widget. False restricts those modifications
- setNoneValue(v)[source]
Sets the new string representation when no model or no model value exists.
- Parameters:
v (str) – the string representation for an invalid value
- setPaused(paused=True)[source]
Toggles the pause mode.
- Parameters:
paused (bool) – whether or not to pause (default = True)
- setShowQuality(showQuality)[source]
Sets/unsets the show quality property
- Parameters:
showQuality (bool) – whether or not to show the quality
- setShowText(showText)[source]
Sets/unsets showing the display value of the model
- Parameters:
showText (bool) – whether or not to show the display value
- setTaurusPopupMenu(**kwargs)
Sets/unsets the taurus popup menu
- param menuData:
an xml representing the popup menu
- type menuData:
str
Deprecated since version 4.5.3a.
- setUseParentModel(**kwargs)
Deprecated since version 4.3.2: Use explicit models including the parent model instead
- property taurusEvent
Base signal taurusEvent