TaurusCommandButton
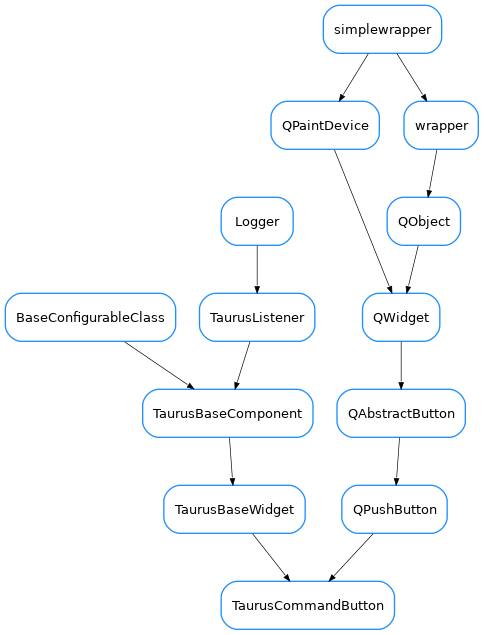
- class TaurusCommandButton(parent=None, designMode=False, command=None, parameters=None, icon=None, text=None, timeout=None)[source]
This class provides a button that executes a tango command on its device.
Code examples:
# a button that executes the "status" command for # the 'a/b/c' device server button = TaurusCommandButton( command = 'Status', icon='logos:taurus.png' ) button.setModel('a/b/c') # a button that executes the "exec" command for # the 'a/b/c' device server with one parameter button = TaurusCommandButton( command = 'Status', parameters=['2+2'],icon='logos:taurus.png' ) button.setModel('a/b/c')
See also
TaurusCommandsForm
provides a good example of use of TaurusCommandButton (including managing the return value)Import from
taurus.qt.qtgui.button
as:from taurus.qt.qtgui.button import TaurusCommandButton
- Command
returns the command name to be executed when the button is clicked
- Returns:
the command name
- Return type:
str or None
- CustomText
Returns the custom text of the buttom, or None if no custom text is used
- DangerMessage
Returns the current apply danger message or None if the apply operation is safe
- Returns:
the apply danger message
- Return type:
str or None
- Model
Returns the model name for this component.
- Parameters
returns the parameters to be used on command execution
- Parameters:
parameters (sequence)
- Timeout
Returns the number of seconds to wait for the result of the command (or -1 if timeout is disabled)
- UseParentModel
(deprecated))
- commandExecuted
- getCommand()[source]
returns the command name to be executed when the button is clicked
- Returns:
the command name
- Return type:
str or None
- getParameters()[source]
returns the parameters to be used on command execution
- Parameters:
parameters (sequence)
- classmethod getQtDesignerPluginInfo()[source]
Returns pertinent information in order to be able to build a valid QtDesigner widget plugin.
The dictionary returned by this method should contain at least the following keys and values:
‘module’ : a string representing the full python module name (ex.: ‘taurus.qt.qtgui.base’)
‘icon’ : a string representing valid resource icon (ex.: ‘designer:combobox.png’)
- ‘container’a bool telling if this widget is a container widget or
not.
This default implementation returns the following dictionary:
{ 'group' : 'Taurus [Unclassified]', 'icon' : 'logos:taurus.png', 'container' : False }
- Returns:
a map with pertinent designer information
- Return type:
- getTimeout()[source]
Returns the number of seconds to wait for the result of the command (or -1 if timeout is disabled)
- setCommand(commandName)[source]
sets the command to be executed when the button is clicked
- Parameters:
commandName (str or None) – the command name
- setCustomText(customText=None)[source]
Sets a custom text for the button (by default it is the command name)
- Parameters:
customText (str or None) – the custom text. If None passed, it will use the command name
- setParameters(parameters)[source]
Sets the parameters to be used on command execution.
- Parameters:
parameters (sequence) – a sequence of parameters. If the elements of the sequence are not of the right type required for the parameter, an automatic conversion will be attempted on execution time. As a special case, if parameters is a string not starting and ending in quote characters, it will be splitted on whitespace to obtain a sequence of parameters. If it is a string starting and ending with quotes, the quotes will be removed and the quoted text will not be splitted.